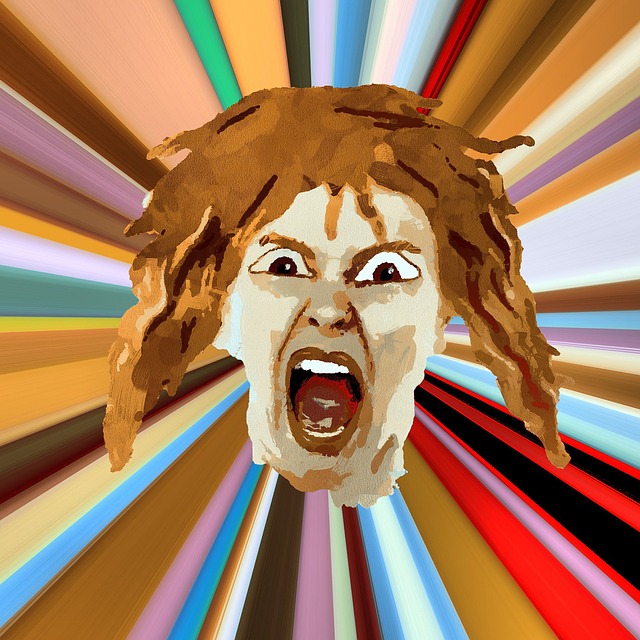
This blog post is for any software engineers who are involved in programming or designing a user interface.
If someone performs a user interface action that should result in an instantaneous and clearly visible response they will only wait patiently for that response for a brief period of time.
If after a five second delay they do not see the expected clearly visible response they will perform the user interface action again, perhaps multiple times if the delay continues for another five seconds or so.
If for some reason there is a delay that prevents the expected instantaneous and clearly visible response, please, for the love of whatever deity or great power you follow, DO NOT PERFORM THE RESPONSE MULTIPLE TIMES.
The multiple user interface actions were not in any way a request to perform the response multiple times. They were multiple increasingly desperate attempts to get the response a single time.
For example, if the user is pressing enter on a desktop icon that starts a program that displays a message box before the main function is even called, they will press enter on the desktop icon multiple times if for some reason it takes between five and sixty seconds to start the application when it normally starts in far less than a second.
Trust me. The user has already started screaming at you by the time they have to hit enter on the desktop icon a third time because the message box still has not been displayed in response to the request to start the application that was made fifteen seconds earlier.
If you then start that application one time for each time they pressed the enter key on the desktop icon when your badly broken operating system finally realizes the user is desperately trying to start it the poor user might end up having a stroke because of the rage they are now experiencing!
Another example is opening a menu such as the start menu or an application menu. Normally this is a very quick operation that takes less than a second. So if five seconds after they pressed the key to open the menu the user does not see the menu, they will press the key to open it again. On the rare occasions when something delays the opening of the menu they may have pressed the open menu key four or five times before it is opened.
That does not mean they want the menu to be opened, then closed, then opened, then closed…
The multiple presses of the key to open the menu were multiple increasingly desperate attempts to open the menu once. By the second attempt the poor user is screaming at you. If you do the open menu, close menu, open menu, close menu, … foolishness it is very likely that the poor user might end up have a stroke because of the rage they are now experiencing!
These are problems I experience very often with Microsoft Windows. To be fair I have seen it with Linux and Mac OSX as well, but no where near as often as I see in in Windows.
Fuck you very much Microsoft for the wonderful user experience of Windows and every single Microsoft product I have every used! If I believed in hell I would wish for everyone responsible for these wonderful user experiences to suffer eternal torment in the hottest and most fiery section of hell!
Thank you for listening to me vent. I feel much better now.
Here is a song that expresses my heartfelt feelings towards Microsoft. Enjoy.